Download this example
Download this example as a Jupyter Notebook or as a Python script.
Import Stackup#
This example shows how to import stackup file.
Import the required packages#
[1]:
import json
from pathlib import Path
import tempfile
from IPython.display import display
from ansys.aedt.core.downloads import download_file
import pandas as pd
from pyedb import Edb
AEDT_VERSION = "2024.2"
NG_MODE = False
C:\Users\ansys\AppData\Local\Temp\ipykernel_5732\2431567430.py:7: DeprecationWarning:
Pyarrow will become a required dependency of pandas in the next major release of pandas (pandas 3.0),
(to allow more performant data types, such as the Arrow string type, and better interoperability with other libraries)
but was not found to be installed on your system.
If this would cause problems for you,
please provide us feedback at https://github.com/pandas-dev/pandas/issues/54466
import pandas as pd
Download the example PCB data.
[2]:
temp_folder = tempfile.TemporaryDirectory(suffix=".ansys")
file_edb = download_file(source="edb/ANSYS-HSD_V1.aedb", destination=temp_folder.name)
Load example layout.#
[3]:
edbapp = Edb(file_edb, edbversion=AEDT_VERSION)
PyAEDT INFO: Logger is initialized in EDB.
PyAEDT INFO: legacy v0.37.0
PyAEDT INFO: Python version 3.10.11 (tags/v3.10.11:7d4cc5a, Apr 5 2023, 00:38:17) [MSC v.1929 64 bit (AMD64)]
PyAEDT INFO: Database ANSYS-HSD_V1.aedb Opened in 2024.2
PyAEDT INFO: Cell main Opened
PyAEDT INFO: Builder was initialized.
PyAEDT INFO: EDB initialized.
Review original stackup definition#
Get original stackup definition in a dictionary. Alternatively, stackup definition can be exported in a json file by edbapp.configuration.export()
[4]:
data_cfg = edbapp.configuration.get_data_from_db(stackup=True)
PyAEDT INFO: Getting data from layout database.
[5]:
df = pd.DataFrame(data=data_cfg["stackup"]["layers"])
display(df)
name | type | material | fill_material | thickness | color | etching | roughness | |
---|---|---|---|---|---|---|---|---|
0 | 1_Top | signal | copper | Solder Resist | 0.035mm | [255, 0, 0] | {'factor': '0', 'enabled': False, 'etch_power_... | {'top': {'model': 'huray', 'nodule_radius': '0... |
1 | DE1 | dielectric | Megtron4 | 0.1mm | [128, 128, 128] | {'factor': '0', 'enabled': False, 'etch_power_... | {'top': {'model': 'huray', 'nodule_radius': '0... | |
2 | Inner1(GND1) | signal | copper | Megtron4_2 | 0.017mm | [128, 128, 0] | {'factor': '0', 'enabled': False, 'etch_power_... | {'top': {'model': 'huray', 'nodule_radius': '0... |
3 | DE2 | dielectric | Megtron4_2 | 0.088mm | [128, 128, 128] | {'factor': '0', 'enabled': False, 'etch_power_... | {'top': {'model': 'huray', 'nodule_radius': '0... | |
4 | Inner2(PWR1) | signal | copper | Megtron4_2 | 0.017mm | [112, 219, 250] | {'factor': '0', 'enabled': False, 'etch_power_... | {'top': {'model': 'huray', 'nodule_radius': '0... |
5 | DE3 | dielectric | Megtron4 | 0.1mm | [128, 128, 128] | {'factor': '0', 'enabled': False, 'etch_power_... | {'top': {'model': 'huray', 'nodule_radius': '0... | |
6 | Inner3(Sig1) | signal | copper | Megtron4_3 | 0.017mm | [255, 0, 255] | {'factor': '0', 'enabled': False, 'etch_power_... | {'top': {'model': 'huray', 'nodule_radius': '0... |
7 | Megtron4-1mm | dielectric | Megtron4_3 | 1mm | [128, 128, 128] | {'factor': '0', 'enabled': False, 'etch_power_... | {'top': {'model': 'huray', 'nodule_radius': '0... | |
8 | Inner4(Sig2) | signal | copper | Megtron4_3 | 0.017mm | [128, 0, 128] | {'factor': '0', 'enabled': False, 'etch_power_... | {'top': {'model': 'huray', 'nodule_radius': '0... |
9 | DE5 | dielectric | Megtron4 | 0.1mm | [128, 128, 128] | {'factor': '0', 'enabled': False, 'etch_power_... | {'top': {'model': 'huray', 'nodule_radius': '0... | |
10 | Inner5(PWR2) | signal | copper | Megtron4_2 | 0.017mm | [0, 204, 102] | {'factor': '0', 'enabled': False, 'etch_power_... | {'top': {'model': 'huray', 'nodule_radius': '0... |
11 | DE6 | dielectric | Megtron4_2 | 0.088mm | [128, 128, 128] | {'factor': '0', 'enabled': False, 'etch_power_... | {'top': {'model': 'huray', 'nodule_radius': '0... | |
12 | Inner6(GND2) | signal | copper | Megtron4_2 | 0.017mm | [0, 128, 128] | {'factor': '0', 'enabled': False, 'etch_power_... | {'top': {'model': 'huray', 'nodule_radius': '0... |
13 | DE7 | dielectric | Megtron4 | 0.1mm | [128, 128, 128] | {'factor': '0', 'enabled': False, 'etch_power_... | {'top': {'model': 'huray', 'nodule_radius': '0... | |
14 | 16_Bottom | signal | copper | Solder Resist | 0.035mm | [0, 0, 255] | {'factor': '0', 'enabled': False, 'etch_power_... | {'top': {'model': 'huray', 'nodule_radius': '0... |
15 | Measures | measure | NaN | NaN | NaN | [63, 63, 63] | NaN | NaN |
16 | SIwave Regions | siwavehfsssolverregions | NaN | NaN | NaN | [127, 127, 127] | NaN | NaN |
17 | Top Overlay | silkscreen | NaN | NaN | NaN | [208, 145, 239] | NaN | NaN |
18 | Top Solder | soldermask | NaN | NaN | NaN | [16, 115, 138] | NaN | NaN |
19 | Bottom Solder | soldermask | NaN | NaN | NaN | [46, 67, 160] | NaN | NaN |
20 | Bottom Overlay | silkscreen | NaN | NaN | NaN | [219, 116, 240] | NaN | NaN |
21 | Outline | outline | NaN | NaN | NaN | [0, 0, 0] | NaN | NaN |
22 | Rats | airlines | NaN | NaN | NaN | [0, 0, 255] | NaN | NaN |
23 | Errors | errors | NaN | NaN | NaN | [255, 0, 0] | NaN | NaN |
24 | Symbols | symbol | NaN | NaN | NaN | [127, 0, 127] | NaN | NaN |
25 | Postprocessing | postprocessing | NaN | NaN | NaN | [40, 152, 137] | NaN | NaN |
Modify stackup#
Modify top layer thickness
[6]:
data_cfg["stackup"]["layers"][0]["thickness"] = 0.00005
Add a solder mask layer
[7]:
data_cfg["stackup"]["layers"].insert(
0, {"name": "soler_mask", "type": "dielectric", "material": "Megtron4", "fill_material": "", "thickness": 0.00002}
)
Review modified stackup
[8]:
df = pd.DataFrame(data=data_cfg["stackup"]["layers"])
display(df.head(3))
name | type | material | fill_material | thickness | color | etching | roughness | |
---|---|---|---|---|---|---|---|---|
0 | soler_mask | dielectric | Megtron4 | 0.00002 | NaN | NaN | NaN | |
1 | 1_Top | signal | copper | Solder Resist | 0.00005 | [255, 0, 0] | {'factor': '0', 'enabled': False, 'etch_power_... | {'top': {'model': 'huray', 'nodule_radius': '0... |
2 | DE1 | dielectric | Megtron4 | 0.1mm | [128, 128, 128] | {'factor': '0', 'enabled': False, 'etch_power_... | {'top': {'model': 'huray', 'nodule_radius': '0... |
Write stackup definition into a json file
[9]:
file_cfg = Path(temp_folder.name) / "edb_configuration.json"
with open(file_cfg, "w") as f:
json.dump(data_cfg, f, indent=4, ensure_ascii=False)
Load stackup from json configuration file#
[10]:
edbapp.configuration.load(file_cfg, apply_file=True)
[10]:
<pyedb.configuration.cfg_data.CfgData at 0x221dc957790>
Plot stackup
[11]:
edbapp.stackup.plot()
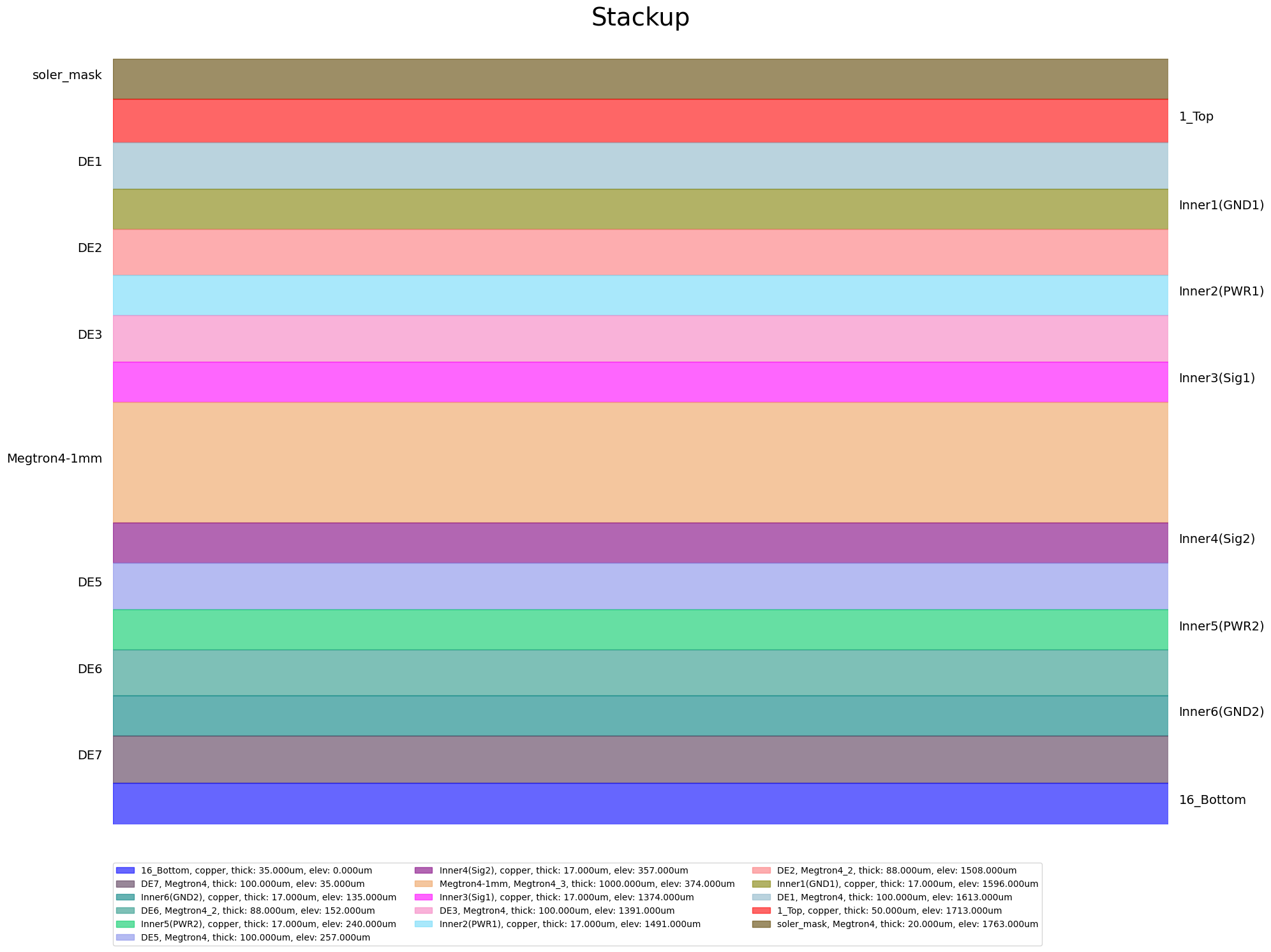
[11]:
<module 'matplotlib.pyplot' from 'C:\\actions-runner\\_work\\pyaedt-examples\\pyaedt-examples\\.venv\\lib\\site-packages\\matplotlib\\pyplot.py'>
Check top layer thickness
[12]:
edbapp.stackup["1_Top"].thickness
[12]:
5e-05
Save and close Edb#
The temporary folder will be deleted once the execution of this script is finished. Replace edbapp.save() with edbapp.save_as(“C:/example.aedb”) to keep the example project.
[13]:
edbapp.save()
edbapp.close()
[13]:
True
Download this example
Download this example as a Jupyter Notebook or as a Python script.