Download this example
Download this example as a Jupyter Notebook or as a Python script.
Circuit schematic creation and analysis#
This example shows how to build a circuit schematic and run a transient circuit simulation.
Keywords: AEDT, Circuit, Schematic.
Import packages and define constants#
Perform required imports.
[1]:
import os
import tempfile
import time
import ansys.aedt.core
Define constants.
[2]:
AEDT_VERSION = "2024.2"
NG_MODE = False # Open AEDT UI when it is launched.
Create temporary directory#
Create a temporary directory where downloaded data or dumped data can be stored. If you’d like to retrieve the project data for subsequent use, the temporary folder name is given by temp_folder.name
.
[3]:
temp_folder = tempfile.TemporaryDirectory(suffix=".ansys")
Launch AEDT with Circuit#
Launch AEDT with Circuit. The pyaedt.Desktop class initializes AEDT and starts the specified version in the specified mode.
[4]:
circuit = ansys.aedt.core.Circuit(
project=os.path.join(temp_folder.name, "CircuitExample"),
design="Simple",
version=AEDT_VERSION,
non_graphical=NG_MODE,
new_desktop=True,
)
circuit.modeler.schematic.schematic_units = "mil"
PyAEDT INFO: Python version 3.10.11 (tags/v3.10.11:7d4cc5a, Apr 5 2023, 00:38:17) [MSC v.1929 64 bit (AMD64)]
PyAEDT INFO: PyAEDT version 0.14.dev0.
PyAEDT INFO: Initializing new Desktop session.
PyAEDT INFO: Log on console is enabled.
PyAEDT INFO: Log on file C:\Users\ansys\AppData\Local\Temp\pyaedt_ansys_96d94839-cee9-4a97-8067-26105a0684c0.log is enabled.
PyAEDT INFO: Log on AEDT is enabled.
PyAEDT INFO: Debug logger is disabled. PyAEDT methods will not be logged.
PyAEDT INFO: Launching PyAEDT with gRPC plugin.
PyAEDT INFO: New AEDT session is starting on gRPC port 51153
PyAEDT INFO: AEDT installation Path C:\Program Files\AnsysEM\v242\Win64
PyAEDT INFO: Ansoft.ElectronicsDesktop.2024.2 version started with process ID 7444.
PyAEDT INFO: Project CircuitExample has been created.
PyAEDT INFO: Added design 'Simple' of type Circuit Design.
PyAEDT INFO: Aedt Objects correctly read
PyAEDT INFO: ModelerCircuit class has been initialized! Elapsed time: 0m 0sec
PyAEDT INFO: ModelerNexxim class has been initialized!
PyAEDT INFO: Modeler class has been initialized! Elapsed time: 0m 0sec
Create circuit setup#
Create and customize a linear network analysis (LNA) setup.
[5]:
setup1 = circuit.create_setup("MyLNA")
setup1.props["SweepDefinition"]["Data"] = "LINC 0GHz 4GHz 10001"
Place components#
Place components such as an inductor, resistor, and capacitor. The location
argument provides the [x, y]
coordinates to place the component.
[6]:
inductor = circuit.modeler.schematic.create_inductor(
name="L1", value=1e-9, location=[0, 0]
)
resistor = circuit.modeler.schematic.create_resistor(
name="R1", value=50, location=[500, 0]
)
capacitor = circuit.modeler.schematic.create_capacitor(
name="C1", value=1e-12, location=[1000, 0]
)
Get all pins#
The component pins are instances of the ansys.aedt.core.modeler.circuits.objct3dcircuit.CircuitPins
class and provide access to the pin location, net connectivity, and the connect_to_component()
method, which can be used to connect components in the schematic as demonstrated in this example.
Place a port and ground#
Place a port and a ground in the schematic.
[7]:
port = circuit.modeler.components.create_interface_port(
name="myport", location=[-300, 50]
)
gnd = circuit.modeler.components.create_gnd(location=[1200, -100])
PyAEDT INFO: Parsing C:/Users/ansys/AppData/Local/Temp/tmprvsxtzc5.ansys/CircuitExample.aedt.
PyAEDT INFO: File C:/Users/ansys/AppData/Local/Temp/tmprvsxtzc5.ansys/CircuitExample.aedt correctly loaded. Elapsed time: 0m 0sec
PyAEDT INFO: aedt file load time 0.0
Connect components#
Connect components with wires in the schematic. The connect_to_component()
method is used to create connections between pins.
[8]:
port.pins[0].connect_to_component(assignment=inductor.pins[0], use_wire=True)
inductor.pins[1].connect_to_component(assignment=resistor.pins[1], use_wire=True)
resistor.pins[0].connect_to_component(assignment=capacitor.pins[0], use_wire=True)
capacitor.pins[1].connect_to_component(assignment=gnd.pins[0], use_wire=True)
[8]:
True
Create transient setup#
Create a transient setup.
[9]:
setup2 = circuit.create_setup(
name="MyTransient", setup_type=circuit.SETUPS.NexximTransient
)
setup2.props["TransientData"] = ["0.01ns", "200ns"]
setup3 = circuit.create_setup(name="MyDC", setup_type=circuit.SETUPS.NexximDC)
Solve transient setup#
Solve the transient setup.
[10]:
circuit.analyze_setup("MyLNA")
circuit.export_fullwave_spice()
PyAEDT INFO: Key Desktop/ActiveDSOConfigurations/Circuit Design correctly changed.
PyAEDT INFO: Solving design setup MyLNA
PyAEDT INFO: Key Desktop/ActiveDSOConfigurations/Circuit Design correctly changed.
PyAEDT INFO: Design setup MyLNA solved correctly in 0.0h 0.0m 6.0s
PyAEDT INFO: FullWaveSpice correctly exported to C:\Users\ansys\AppData\Local\Temp\tmprvsxtzc5.ansys\CircuitExample.pyaedt\Simple\Simple.sp
[10]:
'C:\\Users\\ansys\\AppData\\Local\\Temp\\tmprvsxtzc5.ansys\\CircuitExample.pyaedt\\Simple\\Simple.sp'
Create report#
Create a report displaying the scattering parameters.
[11]:
solutions = circuit.post.get_solution_data(
expressions=circuit.get_traces_for_plot(category="S"),
)
solutions.enable_pandas_output = True
real, imag = solutions.full_matrix_real_imag
print(real)
PyAEDT INFO: Post class has been initialized! Elapsed time: 0m 1sec
PyAEDT INFO: Solution Data Correctly Loaded.
S(myport,myport)
0.0000 1.000000
0.0004 1.000000
0.0008 1.000000
0.0012 0.999999
0.0016 0.999999
... ...
3.9984 0.021101
3.9988 0.021083
3.9992 0.021065
3.9996 0.021046
4.0000 0.021028
[10001 rows x 1 columns]
Create plot#
Create a plot based on solution data.
[12]:
fig = solutions.plot()
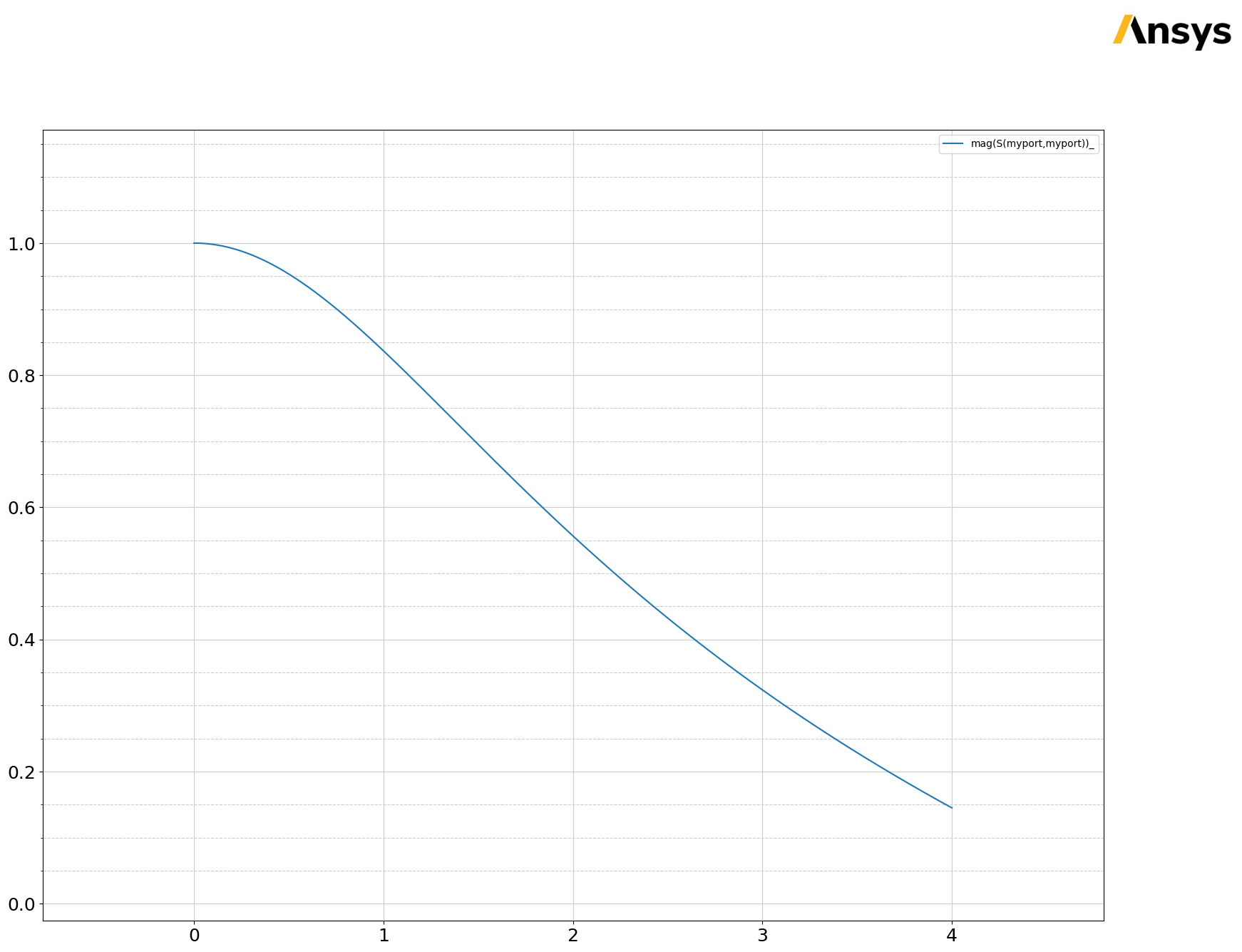
Release AEDT#
Release AEDT and close the example.
[13]:
circuit.save_project()
circuit.release_desktop()
# Wait 3 seconds to allow AEDT to shut down before cleaning the temporary directory.
time.sleep(3)
PyAEDT INFO: Project CircuitExample Saved correctly
PyAEDT INFO: Desktop has been released and closed.
Clean up#
All project files are saved in the folder temp_folder.name
. If you’ve run this example as a Jupyter notebook, you can retrieve those project files. The following cell removes all temporary files, including the project folder.
[14]:
temp_folder.cleanup()
Download this example
Download this example as a Jupyter Notebook or as a Python script.