Download this example
Download this example as a Jupyter Notebook or as a Python script.
Environment import from open street map#
This example shows how to use PyAEDT to create an HFSS SBR+ project from OpenStreetMap.
Keywords: HFSS, SBR+, city, OpenStreetMap
Prerequisites#
Perform imports#
[1]:
import os
import tempfile
import time
import ansys.aedt.core
Define constants#
Constants help ensure consistency and avoid repetition throughout the example.
[2]:
AEDT_VERSION = "2025.1"
NG_MODE = False # Open AEDT UI when it is launched.
Create temporary directory#
Create a temporary working directory. The name of the working folder is stored in temp_folder.name
.
Note: The final cell in the notebook cleans up the temporary folder. If you want to retrieve the AEDT project and data, do so before executing the final cell in the notebook.
[3]:
temp_folder = tempfile.TemporaryDirectory(suffix=".ansys")
Launch HFSS and open project#
Launch HFSS and open the project. The solution type SBR+ instantiates a design type that supports the SBR+ solver.
[4]:
project_name = os.path.join(temp_folder.name, "city.aedt")
app = ansys.aedt.core.Hfss(
project=project_name,
design="Ansys",
solution_type="SBR+",
version=AEDT_VERSION,
new_desktop=True,
non_graphical=NG_MODE,
)
PyAEDT INFO: Python version 3.10.11 (tags/v3.10.11:7d4cc5a, Apr 5 2023, 00:38:17) [MSC v.1929 64 bit (AMD64)].
PyAEDT INFO: PyAEDT version 0.18.dev0.
PyAEDT INFO: Initializing new Desktop session.
PyAEDT INFO: Log on console is enabled.
PyAEDT INFO: Log on file C:\Users\ansys\AppData\Local\Temp\pyaedt_ansys_bf96e22d-88ca-4f0f-a27c-a40b189e1ee6.log is enabled.
PyAEDT INFO: Log on AEDT is disabled.
PyAEDT INFO: Debug logger is disabled. PyAEDT methods will not be logged.
PyAEDT INFO: Launching PyAEDT with gRPC plugin.
PyAEDT INFO: New AEDT session is starting on gRPC port 61157.
PyAEDT INFO: Electronics Desktop started on gRPC port: 61157 after 6.409576654434204 seconds.
PyAEDT INFO: AEDT installation Path C:\Program Files\ANSYS Inc\v251\AnsysEM
PyAEDT INFO: Ansoft.ElectronicsDesktop.2025.1 version started with process ID 10052.
PyAEDT INFO: Project city has been created.
PyAEDT INFO: Added design 'Ansys' of type HFSS.
PyAEDT INFO: Aedt Objects correctly read
Model Preparation#
Define the global location#
Define the latitude and longitude of the location to import from OpenStreetMap.
[5]:
ansys_home = [40.273726, -80.168269]
Generate map and import#
Import the model and define the domain size. The radius that defines the domain is specified in meters.
[6]:
app.modeler.import_from_openstreet_map(
ansys_home,
terrain_radius=250,
road_step=3,
plot_before_importing=True,
import_in_aedt=True,
)
PyAEDT INFO: Modeler class has been initialized! Elapsed time: 0m 0sec
PyAEDT INFO: Generating Terrain
PyAEDT INFO: Terrain Points...
Percent Complete:........................................ 0%
Percent Complete:####.................................... 10%
Percent Complete:########................................ 20%
Percent Complete:############............................ 30%
Percent Complete:################........................ 40%
Percent Complete:####################.................... 50%
Percent Complete:########################................ 60%
Percent Complete:############################............ 70%
Percent Complete:################################........ 80%
Percent Complete:####################################.... 90%
PyAEDT INFO: 100% - Done
PyAEDT INFO: Processing Geometry
PyAEDT INFO: saving STL as C:\Users\ansys\AppData\Local\Temp\tmp64r5v0qk.ansys\city.pyaedt\Ansys/terrain.stl
PyAEDT INFO: Generating Building Geometry
PyAEDT INFO:
Generating Buildings
Percent Complete:######################################## 100.0%
PyAEDT INFO: Generating Road Geometry
C:\actions-runner\_work\pyaedt-examples\pyaedt-examples\.venv\lib\site-packages\pyvista\core\filters\poly_data.py:2997: PyVistaFutureWarning: The default value of the ``capping`` keyword argument will change in a future version to ``True`` to match the behavior of VTK. We recommend passing the keyword explicitly to prevent future surprises.
warnings.warn(
Percent Complete:####.................................... 10.0%
Percent Complete:################........................ 40.0%
Percent Complete:####################.................... 50.0%
Percent Complete:########################................ 60.0%
Percent Complete:####################################.... 90.0%
Percent Complete:######################################## 100.0%
PyAEDT INFO: Done...
PyAEDT INFO: Viewing Geometry...
C:\actions-runner\_work\pyaedt-examples\pyaedt-examples\.venv\lib\site-packages\pyvista\jupyter\notebook.py:37: UserWarning: Failed to use notebook backend:
Please install `ipywidgets`.
Falling back to a static output.
warnings.warn(
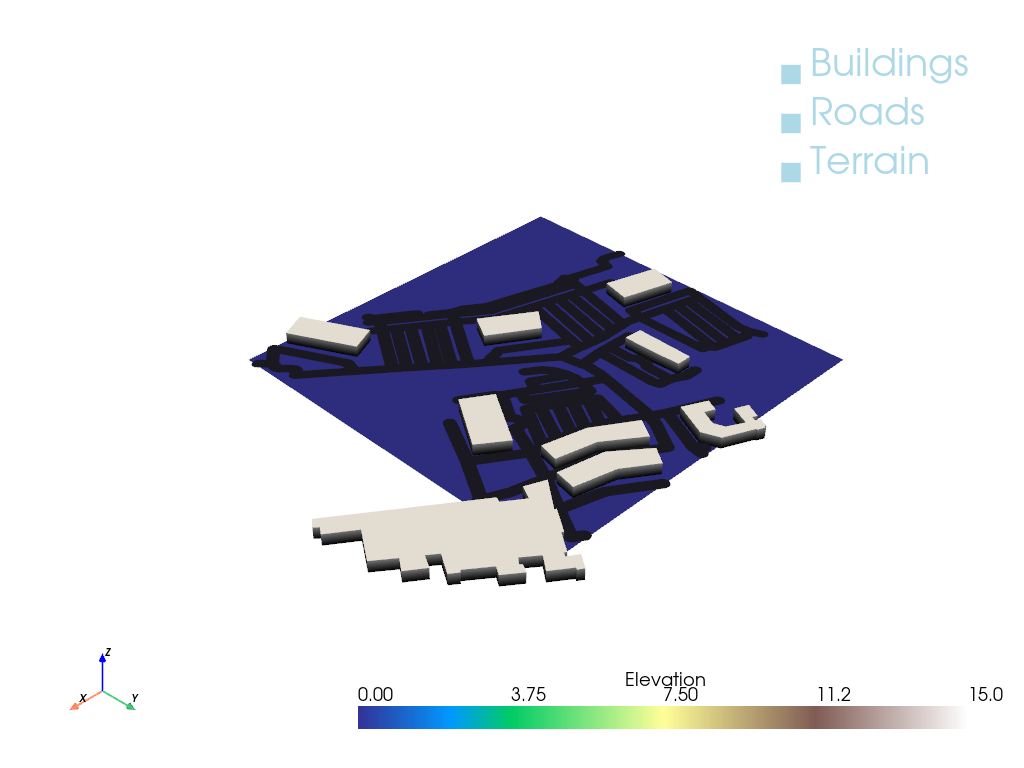
PyAEDT INFO: Step file C:\Users\ansys\AppData\Local\Temp\tmp64r5v0qk.ansys\city.pyaedt\Ansys/terrain.stl imported
PyAEDT INFO: Step file C:\Users\ansys\AppData\Local\Temp\tmp64r5v0qk.ansys\city.pyaedt\Ansys\buildings.stl imported
PyAEDT ERROR: **************************************************************
PyAEDT ERROR: File "C:\Users\ansys\AppData\Local\Programs\Python\Python310\Lib\runpy.py", line 196, in _run_module_as_main
PyAEDT ERROR: return _run_code(code, main_globals, None,
PyAEDT ERROR: File "C:\Users\ansys\AppData\Local\Programs\Python\Python310\Lib\runpy.py", line 86, in _run_code
PyAEDT ERROR: exec(code, run_globals)
PyAEDT ERROR: File "C:\actions-runner\_work\pyaedt-examples\pyaedt-examples\.venv\lib\site-packages\ipykernel_launcher.py", line 18, in <module>
PyAEDT ERROR: app.launch_new_instance()
PyAEDT ERROR: File "C:\actions-runner\_work\pyaedt-examples\pyaedt-examples\.venv\lib\site-packages\traitlets\config\application.py", line 1075, in launch_instance
PyAEDT ERROR: app.start()
PyAEDT ERROR: File "C:\actions-runner\_work\pyaedt-examples\pyaedt-examples\.venv\lib\site-packages\ipykernel\kernelapp.py", line 739, in start
PyAEDT ERROR: self.io_loop.start()
PyAEDT ERROR: File "C:\actions-runner\_work\pyaedt-examples\pyaedt-examples\.venv\lib\site-packages\tornado\platform\asyncio.py", line 211, in start
PyAEDT ERROR: self.asyncio_loop.run_forever()
PyAEDT ERROR: File "C:\Users\ansys\AppData\Local\Programs\Python\Python310\Lib\asyncio\base_events.py", line 603, in run_forever
PyAEDT ERROR: self._run_once()
PyAEDT ERROR: File "C:\Users\ansys\AppData\Local\Programs\Python\Python310\Lib\asyncio\base_events.py", line 1909, in _run_once
PyAEDT ERROR: handle._run()
PyAEDT ERROR: File "C:\Users\ansys\AppData\Local\Programs\Python\Python310\Lib\asyncio\events.py", line 80, in _run
PyAEDT ERROR: self._context.run(self._callback, *self._args)
PyAEDT ERROR: File "C:\actions-runner\_work\pyaedt-examples\pyaedt-examples\.venv\lib\site-packages\ipykernel\kernelbase.py", line 545, in dispatch_queue
PyAEDT ERROR: await self.process_one()
PyAEDT ERROR: File "C:\actions-runner\_work\pyaedt-examples\pyaedt-examples\.venv\lib\site-packages\ipykernel\kernelbase.py", line 534, in process_one
PyAEDT ERROR: await dispatch(*args)
PyAEDT ERROR: File "C:\actions-runner\_work\pyaedt-examples\pyaedt-examples\.venv\lib\site-packages\ipykernel\kernelbase.py", line 437, in dispatch_shell
PyAEDT ERROR: await result
PyAEDT ERROR: File "C:\actions-runner\_work\pyaedt-examples\pyaedt-examples\.venv\lib\site-packages\ipykernel\ipkernel.py", line 362, in execute_request
PyAEDT ERROR: await super().execute_request(stream, ident, parent)
PyAEDT ERROR: File "C:\actions-runner\_work\pyaedt-examples\pyaedt-examples\.venv\lib\site-packages\ipykernel\kernelbase.py", line 778, in execute_request
PyAEDT ERROR: reply_content = await reply_content
PyAEDT ERROR: File "C:\actions-runner\_work\pyaedt-examples\pyaedt-examples\.venv\lib\site-packages\ipykernel\ipkernel.py", line 449, in do_execute
PyAEDT ERROR: res = shell.run_cell(
PyAEDT ERROR: File "C:\actions-runner\_work\pyaedt-examples\pyaedt-examples\.venv\lib\site-packages\ipykernel\zmqshell.py", line 549, in run_cell
PyAEDT ERROR: return super().run_cell(*args, **kwargs)
PyAEDT ERROR: File "C:\Users\ansys\AppData\Local\Temp\ipykernel_10444\2461479977.py", line 1, in <module>
PyAEDT ERROR: app.modeler.import_from_openstreet_map(
PyAEDT ERROR: File "C:\actions-runner\_work\pyaedt-examples\pyaedt-examples\.venv\lib\site-packages\ansys\aedt\core\modeler\modeler_3d.py", line 1340, in import_from_openstreet_map
PyAEDT ERROR: self.import_3d_cad(parts_dict[part]["file_name"], create_lightweigth_part=create_lightweigth_part)
PyAEDT ERROR: File "C:\actions-runner\_work\pyaedt-examples\pyaedt-examples\.venv\lib\site-packages\ansys\aedt\core\modeler\cad\primitives.py", line 4995, in import_3d_cad
PyAEDT ERROR: self.oeditor.Import(vArg1)
PyAEDT ERROR: AEDT API Error on import_3d_cad
PyAEDT ERROR: Last Electronics Desktop Message - [error] import native bodies: translation of c:/users/ansys/appdata/local/temp/tmp64r5v0qk.ansys/city.pyaedt/ansys/roads.stl failed. (04:59:43 am jun 28, 2025)
PyAEDT ERROR: Method arguments:
PyAEDT ERROR: input_file = C:\Users\ansys\AppData\Local\Temp\tmp64r5v0qk.ansys\city.pyaedt\Ansys\roads.stl
PyAEDT ERROR: create_lightweigth_part = True
PyAEDT ERROR: **************************************************************
[6]:
{'name': 'default',
'version': 1,
'type': 'environment',
'center_lat_lon': [40.273726, -80.168269],
'radius': 250,
'include_buildings': True,
'include_roads': True,
'parts': {'terrain': {'file_name': 'C:\\Users\\ansys\\AppData\\Local\\Temp\\tmp64r5v0qk.ansys\\city.pyaedt\\Ansys/terrain.stl',
'color': 'brown',
'material': 'earth'},
'buildings': {'file_name': 'C:\\Users\\ansys\\AppData\\Local\\Temp\\tmp64r5v0qk.ansys\\city.pyaedt\\Ansys\\buildings.stl',
'color': 'grey',
'material': 'concrete'},
'roads': {'file_name': 'C:\\Users\\ansys\\AppData\\Local\\Temp\\tmp64r5v0qk.ansys\\city.pyaedt\\Ansys\\roads.stl',
'color': 'black',
'material': 'asphalt'}}}
Visualize the model#
Plot the model.
[7]:
plot_obj = app.plot(show=False, plot_air_objects=True)
plot_obj.background_color = [153, 203, 255]
plot_obj.zoom = 1.5
plot_obj.show_grid = False
plot_obj.show_axes = False
plot_obj.bounding_box = False
plot_obj.plot(os.path.join(temp_folder.name, "Source.jpg"))
PyAEDT INFO: Parsing C:/Users/ansys/AppData/Local/Temp/tmp64r5v0qk.ansys/city.aedt.
PyAEDT INFO: File C:/Users/ansys/AppData/Local/Temp/tmp64r5v0qk.ansys/city.aedt correctly loaded. Elapsed time: 0m 0sec
PyAEDT INFO: aedt file load time 0.016750097274780273
PyAEDT INFO: PostProcessor class has been initialized! Elapsed time: 0m 0sec
PyAEDT INFO: PostProcessor class has been initialized! Elapsed time: 0m 0sec
PyAEDT INFO: Post class has been initialized! Elapsed time: 0m 0sec
C:\actions-runner\_work\pyaedt-examples\pyaedt-examples\.venv\lib\site-packages\pyvista\jupyter\notebook.py:37: UserWarning: Failed to use notebook backend:
Please install `ipywidgets`.
Falling back to a static output.
warnings.warn(
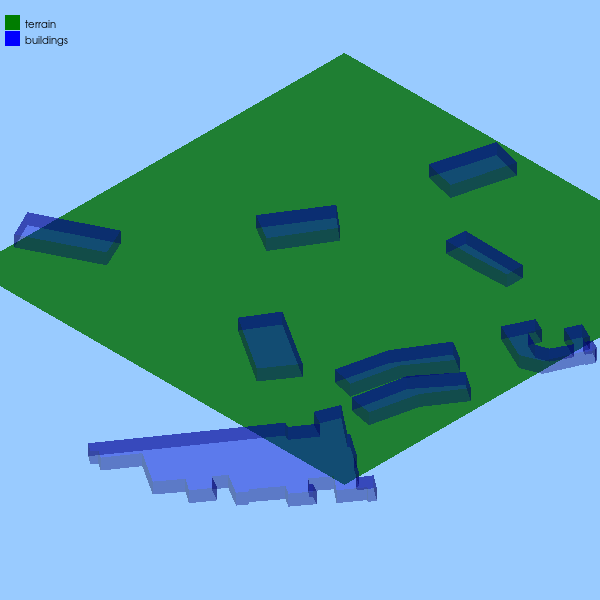
[7]:
True
Finish#
Save the project#
Release AEDT and close the example.
[8]:
app.save_project()
app.release_desktop()
# Wait 3 seconds to allow AEDT to shut down before cleaning the temporary directory.
time.sleep(3)
PyAEDT INFO: Project city Saved correctly
PyAEDT INFO: Desktop has been released and closed.
Clean up#
All project files are saved in the folder temp_folder.name
. If you’ve run this example as a Jupyter notebook, you can retrieve those project files. The following cell removes all temporary files, including the project folder.
[9]:
temp_folder.cleanup()
Download this example
Download this example as a Jupyter Notebook or as a Python script.